Delete
In order to make changes we will add the code for mnuDelete, mnuAdd, and mnuEdit.
Add and Edit use the list box form.
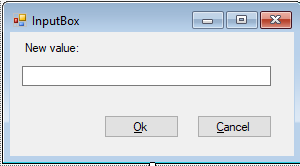
Write the code for mnuDelete and mnuSaveAs as shown below.
private void mnuDelete_Click(object sender, EventArgs e)
{
// Remove the selected item from the list box.
lstData.Items.Remove(lstData.SelectedItem);
changed = true;
}
private void mnuAdd_Click(object sender, EventArgs e)
{
// Display the input box form to let the user type in a new item.
InputBox box = new InputBox();
box.ShowDialog();
TextBox txt = box.getTextBox();
// This gives us accfess to the text box on the form.
if (txt.Text != "")
{
// If a new item was typed, add it to list box.
lstData.Items.Add(txt.Text);
changed = true;
}
}
private void mnuEdit_Click(object sender, EventArgs e)
{
// Display the input box form to let the user edit the current item.
InputBox box = new InputBox();
TextBox txt = box.getTextBox();
// Put the current item into the text box to edit.
txt.Text = lstData.Text;
box.ShowDialog();
// Save the current index of list box, so that old item
// can be deleted and new item inserted in the same position.
int pos = lstData.SelectedIndex;
lstData.Items.Remove(lstData.SelectedItem);
// If they changed the text to "", assume it is to be deleted.
if (txt.Text != "") lstData.Items.Insert(pos, txt.Text);
changed = true;
}
To Do: Make sure this works before proceeding.