Regular Expressions
A regular expression is a way to determine if a string matches a pattern.
Regular Expressions
Regular expressions can be used to validate strings.
A regular expression is a way to determine if a string matches a pattern.
The regular expression syntax can be rather complex.
We will start with a fairly simple example: whether a string is a valid Social Security number.
Modify the social security program from the earlier demo.
: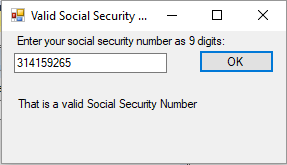
For now we will assume that the only valid string is 9 digits.
We can represent that pattern as [0-9]{9} meaning that an element in the set of digits [0-9] must be repeated {9} times. This expression does not allow any dashes.
Notice that we must import regular expressions: insert the line below after the other "using" directives at the top of the code.
using System.Text.RegularExpressions;
...
private void btnOK_Click(object sender, EventArgs e)
{
String s = txtInput.Text;
Regex socregx= new Regex("[0-9]{9}");
Match m = socregx.Match(s);
if (m.Value==s) lblOutput.Text = "OK";
else lblOutput.Text = "Not valid";
}
We could use "[0-9]{3}-{0,1}[0-9]{2}-{0,1}[0-9]{4}" to accept the dashes. This says that there must be 3 digits followed by 0 or 1 dashes, followed by 2 digits, followed by 0 or 1 dashes, followed by 4 digits.