There are several ways to validate a string. In the example below we have a string that should be a Social Security Number.
It should have a length of 9 and consist entirely of digits.
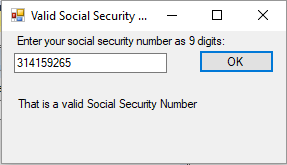
Build the form as shown:
- There is a label
lblInstructions with the words "Enter your social security number as 9 digits:"
- There is a text box
named txtInput.
- There is a button
, btnOK.
- There is a label
named lblOutput.
- Set the accept button for the form to btnOK.
Test various different values. Try enough variations so that you are sure it works.
private void btnOK_Click(object sender, EventArgs e)
{
// Check if the input is a valid Social Security number, ie. 9 digits.
String s = txtInput.Text;
// Assume the string is valid until you find it to be invalid.
Boolean ok = true;
if (s.Length != 9) ok = false;
else
{
// Convert the string to an array of characters.
char[] chars = s.ToCharArray();
// Check that each character is a digit.
for(int i=0;i<9;i++)
{
if (chars[i] < '0' || chars[i] > '9') ok = false;
}
}
// Display the results.
if (ok) lblOutput.Text = "That is a valid Social Security Number";
else lblOutput.Text = "That is NOT a valid Social Security Number";
}
}
Experiment: How would you allow the 2 dashes: 314-15-9265?
How would you make it accept either with or without the dashes: 314159265 or 314-15-9265?
End of lesson, Next lesson: Files in C#